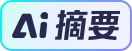
nginx+纯PHP8.0+SQLite数据库运行,仅管理员登陆使用+添加短网址自定义路径,默认4位随机数(字母+数字),添加后支持修改标题+长链接。
扩展功能,添加其它域名,快速添加(登陆后,首页前端输入长链接直接生产短网址支持批量生成)
数据统计,访问量(今天昨天30天,单网址总访问量)-添加时间-到期时间。
打开方法:不限制打开方式,微信内打开,手机浏览器打开,电脑浏览器打开,微信QQ防红图片引导浏览器打开,暂停打开。
首页需要添加备案号
安装流程:访问域名,进入安装页面,填写管理员名称+密码,进入后台登陆页面,登陆使用。
1.安装页面(install.php):
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>安装</title>
</head>
<body>
<h1>安装</h1>
<form action="install.php" method="post">
<label for="username">管理员名称:</label>
<input type="text" name="username" id="username" required><br>
<label for="password">密码:</label>
<input type="password" name="password" id="password" required><br>
<input type="submit" value="安装">
</form>
</body>
</html>
2.安装处理(install.php):
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$username = $_POST['username'];
$password = $_POST['password'];
// 连接SQLite数据库
$db = new SQLite3('short_url.db');
$db->exec('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, username TEXT, password TEXT)');
// 添加管理员账户
$hashed_password = password_hash($password, PASSWORD_DEFAULT);
$db->exec("INSERT INTO users (username, password) VALUES ('$username', '$hashed_password')");
// 跳转到后台登录页面
header('Location: login.php');
exit;
}
?>
3.后台登录页面(login.php):
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>登录</title>
</head>
<body>
<h1>登录</h1>
<form action="login.php" method="post">
<label for="username">用户名:</label>
<input type="text" name="username" id="username" required><br>
<label for="password">密码:</label>
<input type="password" name="password" id="password" required><br>
<input type="submit" value="登录">
</form>
</body>
</html>
4.登录处理(login.php):
<?php
session_start();
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$username = $_POST['username'];
$password = $_POST['password'];
// 连接SQLite数据库
$db = new SQLite3('short_url.db');
// 验证用户名和密码
$result = $db->query("SELECT * FROM users WHERE username='$username'");
if ($result && $row = $result->fetchArray(SQLITE3_ASSOC)) {
if (password_verify($password, $row['password'])) {
$_SESSION['user_id'] = $row['id'];
header('Location: admin.php');
exit;
} else {
echo '密码错误';
}
} else {
echo '用户名不存在';
}
}
?>
后台管理页面(admin.php):
<?php
session_start();
if (!isset($_SESSION['user_id'])) {
header('Location: login.php');
exit;
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>管理</title>
</head>
<body>
<h1>管理</h1>
<!-- 添加短网址表单 -->
<form action="add_short_url.php" method="post">
<label for="long_url">长链接:</label>
<input type="text" name="long_url" id="long_url" required><br>
<label for="custom_path">自定义路径:</label>
<input type="text" name="custom_path" id="custom_path"><br>
<label for="title">标题:</label>
<input type="text" name="title" id="title"><br>
<input type="submit" value="添加短网址">
</form>
<!-- 批量添加短网址表单 -->
<form action="batch_add_short_urls.php" method="post">
<label for="long_urls">长链接列表(每行一个):</label><br>
<textarea name="long_urls" id="long_urls" rows="10" cols="30"></textarea><br>
<input type="submit" value="批量添加短网址">
</form>
</body>
</html>
添加短网址处理(add_short_url.php):
<?php
session_start();
if (!isset($_SESSION['user_id'])) {
header('Location: login.php');
exit;
}
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$long_url = $_POST['long_url'];
$custom_path = $_POST['custom_path'];
$title = $_POST['title'];
// 连接SQLite数据库
$db = new SQLite3('short_url.db');
// 生成短网址
$short_url = generate_short_url($long_url, $custom_path);
// 添加短网址记录
$add_time = time();
$expire_time = strtotime('+30 days', $add_time);
$visit_count = 0;
$db->exec("INSERT INTO urls (long_url, short_url, title, add_time, expire_time, visit_count) VALUES ('$long_url', '$short_url', '$title', $add_time, $expire_time, $visit_count)");
// 跳转回管理页面
header('Location: admin.php');
exit;
}
?>
批量添加短网址处理(batch_add_short_urls.php):
<?php
session_start();
if (!isset($_SESSION['user_id'])) {
header('Location: login.php');
exit;
}
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$long_urls = explode("\n", $_POST['long_urls']);
$custom_path = $_POST['custom_path'];
$title = $_POST['title'];
// 连接SQLite数据库
$db = new SQLite3('short_url.db');
foreach ($long_urls as $long_url) {
// 生成短网址
$short_url = generate_short_url($long_url, $custom_path);
// 添加短网址记录
$add_time = time();
$expire_time = strtotime('+30 days', $add_time);
$visit_count = 0;
$db->exec("INSERT INTO urls (long_url, short_url, title, add_time, expire_time, visit_count) VALUES ('$long_url', '$short_url', '$title', $add_time, $expire_time, $visit_count)");
}
// 跳转回管理页面
header('Location: admin.php');
exit;
}
?>
本文来自投稿,不代表本站立场,如若转载,请注明出处: